Android - Integrating the SDK
How to integrate the Android Voice SDK
SDK Setup
Requirements
To use the Voice SDK you need:
- The latest version of Android Studio
- Android SDK Version 26 or above
- An Android device compatible with Google Play Services
Setup the development environment
Complete the following steps to setup your Android studio development environment:
- Open Android Studio and click Start a new Android Studio project.
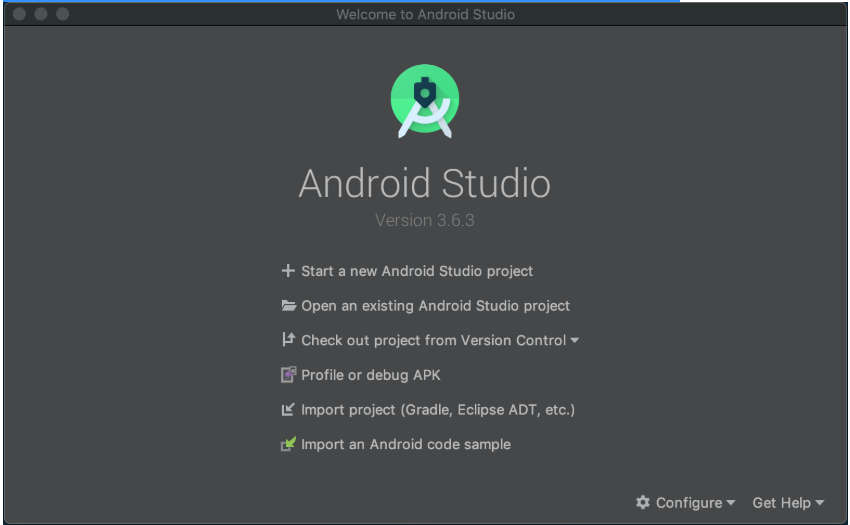
Android Studio 1
- In the Select a Project Template window, select a template of your choice and click Next.
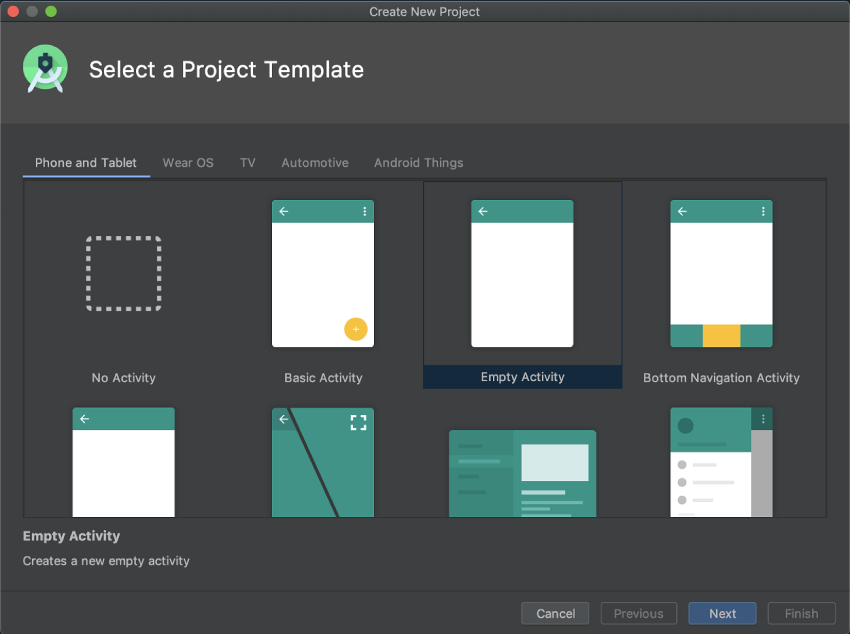
Android Studio 2
-
In the Configure Your Project window:
- Enter the name of your app and your Package name.
- Enter the location of where your project will be saved
- Select either Kotlin or Java as the language
- Select API 26: Android 8.0 (Oreo) as the Minimum SDK and click Finish.
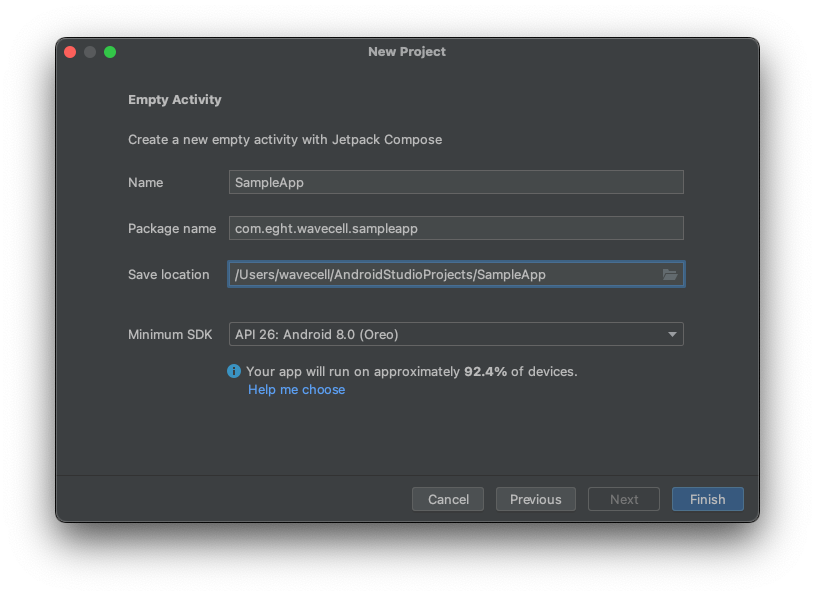
Android Studio 3
Note:: The Voice SDK APIs expose Kotlin coroutine APIs such as Flows and
suspend
functions. The easiest way to consume such APIs from Java is to use RxJava in conjunction with coroutine to Rx extensions.
Add the Voice SDK as a dependency
Confirm you have the latest dependency version:
- Add the following to your top-level
build.gradle
file:
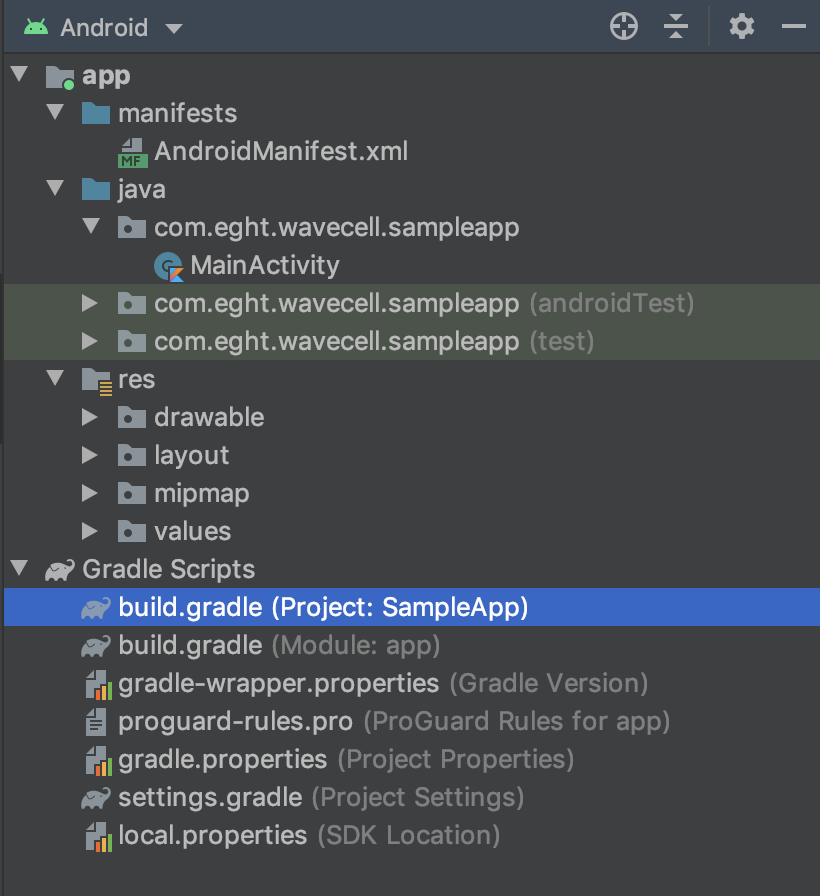
Android Studio 4
allprojects {
repositories {
...
maven {
url "https://github.com/8x8Cloud/voice-sdk-android/raw/master/releases/"
}
}
}
- Add the Wavecell dependency as well as
sourceCompatibility
in your app-levelbuild.gradle
file:
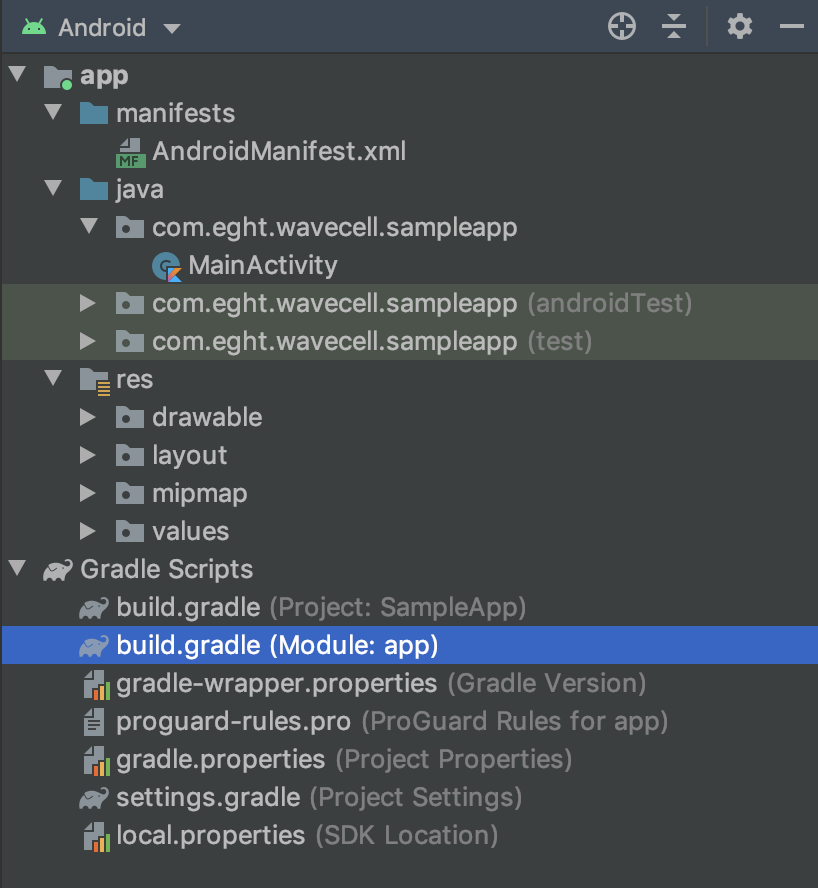
Android Studio 5
android {
...
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
dependencies {
...
implementation "com.eght:wavecell-voice-sdk:[LATEST_VERSION]"
}
- Click on File > Sync Project with Gradle Files.
You can now use the Voice SDK in your application.
Permissions
Add the following uses-permission
elements to your AndroidManifest
:
<manifest>
...
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<uses-permission android:name="android.permission.READ_PHONE_STATE" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
</manifest>
If your application is targeting API level 31+ also include the
<uses-permission android:name="android.permission.READ_PHONE_NUMBERS" />
Updated almost 2 years ago
What’s Next